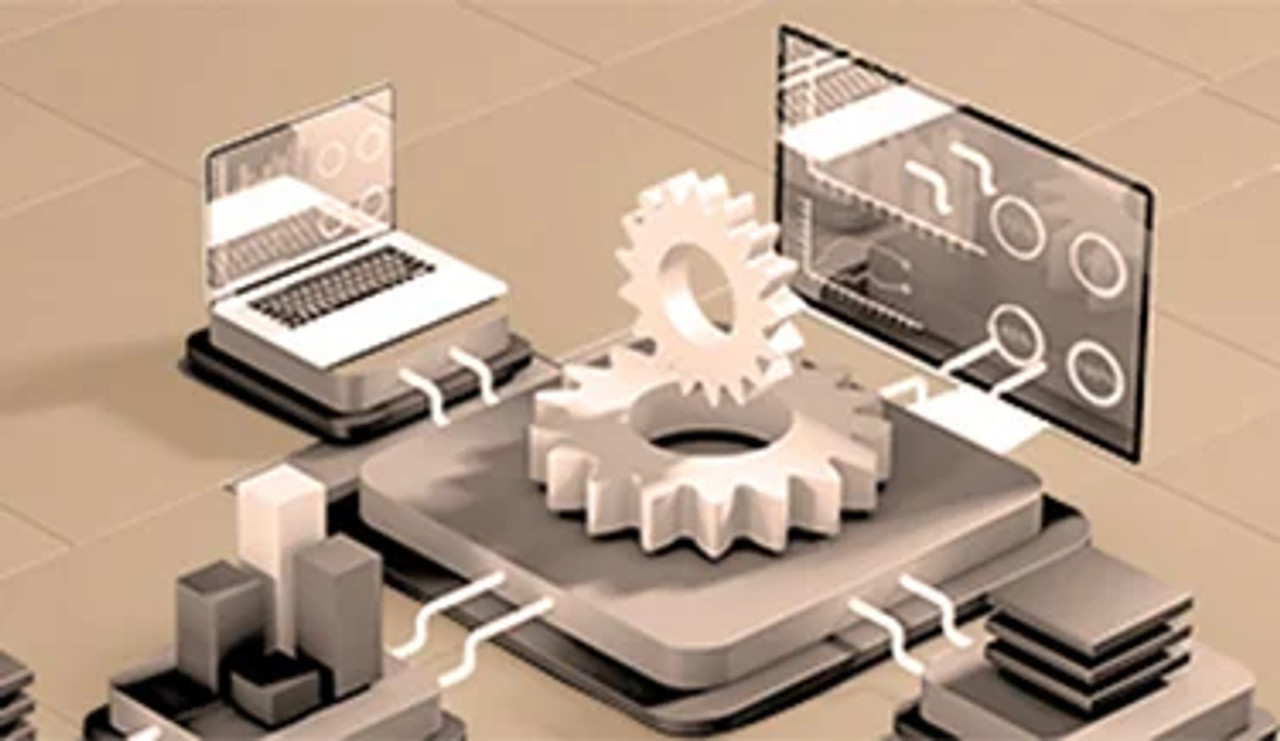
$99
Plus membership
3 Credits
All courses include:
eTextbooks
2 to 3-day turnaround for grading
Multiple chances to improve your grade
On-demand tutoring & writing center
Student support 7 days a week
$99
Plus membership
3 Credits
All courses include:
eTextbooks
2 to 3-day turnaround for grading
Multiple chances to improve your grade
On-demand tutoring & writing center
Student support 7 days a week
Introduction to Programming - C++
$99
Plus membership
3 Credits
About This Course
ACE Approved 2023
In our Introduction to Programming online course, choose one of three programming languages you want to learn C++, Python, or Java. Regardless of which language you select, you will also learn about the core computer science concepts of variables, branching, loops, arrays/lists, and functions/methods. Intro to Programming teaches you object-oriented programming, covers use pointers and streams, and provides a variety of good coding practices, including iterative development, code formatting, and variable naming schemes.
Course Outcomes
Use standard input and output, and understand common syntax errors
Declare and initialize variables with valid identifiers
Develop programs that branch based on user input
Combine loops and arrays/lists, and develop programs with multiple arrays/lists
Write a function/method, then return from a function/method and parameterize a function
Initialize class variables with class constructor
Create derived and abstract classes
Write a recursive function
Use binary search, O notation, and algorithm analysis
Course Text
Prefer the hard copy? Simply purchase from your favorite textbook reseller; you will still get the eTextbook for free. There is no text for this course. All materials are included in the course fee.
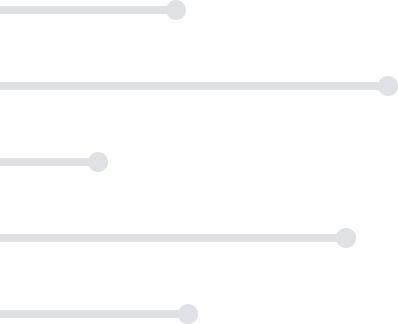
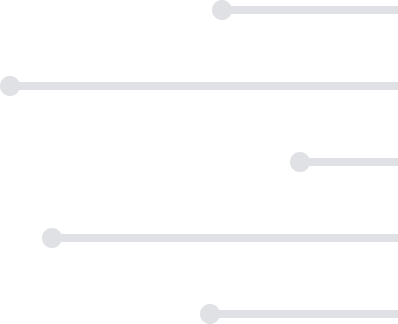
Earn College Credit That Will Transfer
Transfer into over 3000+ institutions that accept ACE courses or transfer directly into 150+ partner schools.
view all partners